In the previous post we set up the Salesforce Code Analyzer to run several static code analyses from a single tool.
Salesforce Graph Engine performs more complex checks than an average static analysis tool because Graph Engine uses data flow analysis, which is a technique for gathering information about the possible set of values calculated at various points in the application. If you’ve worked with other IDEs, such as IntelliJ, then you will be familiar with this type of data flow code analysis.
Salesforce Graph Engine brings support for catching security and quality issues that can’t be detected with static code analysis alone, including null pointer exceptions.
In this post we will look at how to use Salesforce Graph Engine to detect potential null pointer exceptions, a very common and easy-to-introduce bug that can cause errors at runtime.
Prerequisites
- Complete the steps for installing Salesforce Code Analyzer
Using Salesforce Graph Engine
Open a project in DX format with VS Code, or navigate to the root of a DX project in your terminal. Alternatively you can use the the sample app provided by the Code Analyzer team.
Graph Engine Rules
First let’s see the list of Graph Engine rules included in the current release
sf scanner rule list --engine sfge
The –engine flag can be used to only list the rules related to a specific engine, such as pmd, eslint-typescript, graph engine, and others.
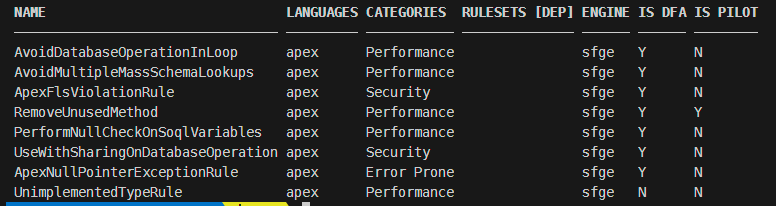
Data Flow Analysis Entry Points
Keep in mind that the graph engine analyzes code execution paths only from certain entry points. These entry points will vary somewhat by rule. These are the entry paths for the Null Pointer Exception rule:
- @AuraEnabled-annotated methods
- @InvocableMethod-annotated methods
- @NamespaceAccessible-annotated methods
- @RemoteAction-annotated methods
- Any method returning a PageReference object
- public-scoped methods on Visualforce Controllers
- global-scoped methods on any class
- Messaging.InboundEmailResult handleInboundEmail() methods on implementations of Messaging.InboundEmailHandler
- Any method targeted during invocation
So the Graph Engine won’t scan code for null pointer exceptions that isn’t referenced by a class that falls into one of the above categories.
Scan with Graph Engine
Now lets test the null pointer exception rule. Create a new Apex class called WeatherService.cls and add the following code
public with sharing class WeatherService {
@AuraEnabled
public static string convertCelcius(){
try {
int celcius;
int fahrenheit = celcius * 9 / 5 + 32;
return fahrenheit;
} catch (Exception e) {
throw new AuraHandledException(e.getMessage());
}
}
}
Run the scan on a specific file
sf scanner run dfa --target './force-app/main/default/classes/WeatherService.cls' --projectdir './force-app/main/default' --format html > sfge_scan_results.html
Open the sfge_scan_result.html file in your browser to view the report.
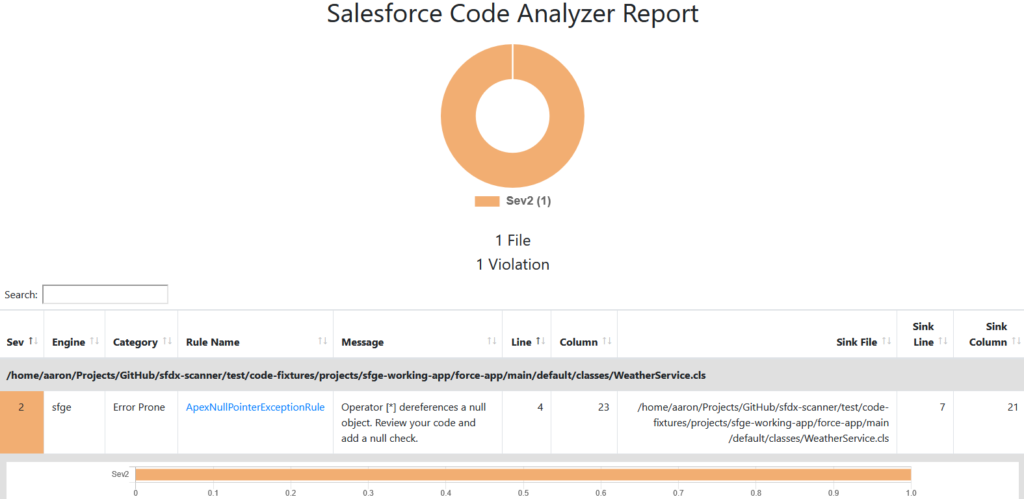
You can also run the scan against the entire project to view all issues caught by the data flow analysis and browser or search through the report.
sf scanner run dfa --target './force-app/main/default/classes' --projectdir './force-app/main/default' --format html > sfge_scan_results.html
The Salesforce Code Analyzer can output results into a number of formats depending on your use case. Use the –format option with one of the values below. Note that the output is sent directly to the console so you may want to send the results to a file that can be used for reporting, or integration with the CI/CD pipeline, etc.
-f, --format=(csv|html|json|junit|sarif|table|xml)
Advanced Topics
If you are working on a large, complex code base, you may run into Graph Engine limits, and you may need to allocate more memory to the Graph Engine, or look into refactoring the code entry points to simplify the amount of analysis required by the Graph Engine.
Sometimes you may need to disable a rule from scanning a particular method or line of code. The Graph Engine supports this requirement through structured comments.
Summary
This post explored how to use the Salesforce Graph Engine to conduct data flow analysis and uncover quality and security issues not identifiable through normal static code analysis. Salesforce Graph Engine is provided with the Salesforce Code Analyzer, a plugin to the Salesforce CLI. While the tooling is still in early development, it is worth exploring as there is a lot of potential benefit.
Resources
- Documentation: Salesforce Code Analyzer Command Reference
- Documentation: Current Graph Engine Rules
- Documentation: Graph Engine Limits
- Source Code: Salesforce Code Analyzer Project